Storages in Android:Shared Preferences
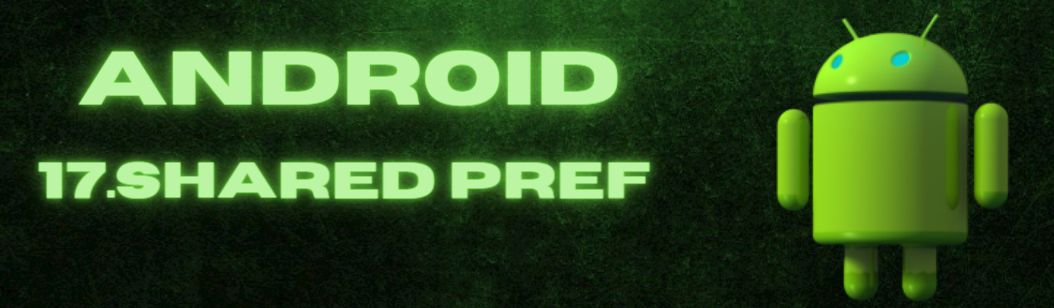
In Android, storage refers to the various ways in which data can be saved and retrieved on a device.
Saving data makes apps livelier
Data persistence = Storing & Saving the data
In android, we can save data using these common ways:
- Text file – (Not Efficient)
- Shared Preferences – (Somewhat Efficient)
- Database – (Highly Efficient)
Table of Contents
Shared Preferences
Shared preferences is a lightweight storage mechanism provided by the Android framework. It enables apps to store primitive data types in key-value pairs, making it suitable for storing small amounts of data, app settings, and user preferences.
Shared Preferences allows activities and applications to keep preferences, in the form of key-value pairs similar to a Map that will persist even when the user closes the application.
Android stores Shared Preferences settings as XML file in shared_prefs folder under
DATA/data/{application package} directory.
The DATA folder can be obtained bycalling Environment.getDataDirectory().
SharedPreferences is application specific,
i.e. the data is lost on performing one of the following options:
on uninstalling the application
on clearing the application data (through Settings)
Shared Preferences can be thought of as a dictionary or a key/value pair.
for example, you might have a key being “username” and for the value, you might store the user’s username. And then you could retrieve that by its key (here username).
You can have a simple shared preference API that you can use to store preferences and pull them back as and when needed.
Shared Preferences class provides APIs for reading, writing, and managing this data.
Example
In this app whatever data enter in editText is display on the textView.
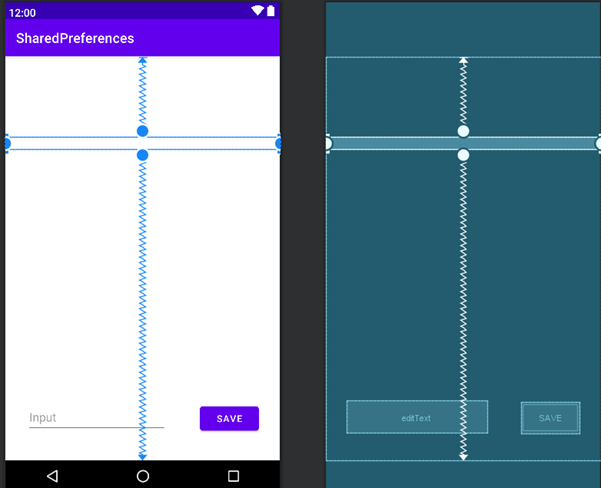
Kotlin:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val textView = findViewById<TextView>(R.id.textView)
val btn = findViewById<Button>(R.id.button)
val edt = findViewById<EditText>(R.id.editText)
btn.setOnClickListener {
val msg = edt.text.toString()
val shrd = getSharedPreferences("MyPref", MODE_PRIVATE)
val editor = shrd.edit()
editor.putString("Message", msg)
editor.apply()
textView.text = msg
}
val getShared = getSharedPreferences("MyPref", MODE_PRIVATE)
val str = getShared.getString("Message", "Save a note and show it here")
textView.text = str
}
}
Java:

Get a handle to shared preferences
You can create a new shared preference file or access an existing one by calling one of these methods:
getPreferences() : used from within your Activity, to access activity-specific preferences.
getSharedPreferences() : used from within your Activity (or other application Context), to access application-level preferences.
getDefaultSharedPreferences() : used on the PreferenceManager, to get the shared preferences that work in concert with Android’s overall preference framework.
In this the method is defined asfollows:
getSharedPreferences (String PREFS_NAME, int mode)
PREFS_NAME is the name of the file. mode is the operating mode. Following are the operating modes applicable:
MODE_PRIVATE: the default mode, where the created file can only be accessed by the calling MODE_WORLD_READABLE: Creating world-readable files is very dangerous, and likely to cause security holes in applications.
MODE_WORLD_WRITEABLE: Creating world-writable files is very dangerous, and likely to
cause security holes in applications
MODE_MULTI_PROCESS: This method will check for modification of preferences even if the
Shared Preference instance has already been loaded
MODE_APPEND: This will append the new preferences with the already existing preferences
MODE_ENABLE_WRITE_AHEAD_LOGGING: Database open flag. When it is set, it would
enable write ahead logging by default
Write to shared preferences
To write to a shared preferences file, create a SharedPreferences.Editor by calling edit() on your SharedPreferences
Pass the keys and values you want to write with methods such as putInt() and putString(). Then call apply() or commit() to save the changes.
Whatever value in editText is stored in SharedPreferences corresponding to key “Message” and value msg.
apply() changes the in-memory SharedPreferences object immediately but writes the updates to disk asynchronously.
Alternatively, you can use commit() to write the data to disk synchronously (.commit() will stored preferences in this exact movement).
But because commit() is synchronous, you should avoid calling it from your main thread because it could pause your UI rendering.
Read from shared preferences
To retrieve values from a shared preferences file, call methods such as getInt() and getString(), providing the key for the value you want, and optionally a default value to return if the key isn’t present.

Default value:”Save a note and show it here”
If we Clear cache and clear the storage of App then this data is deleted.
App with Switch Component and SharedPreferences
If switch is turned ON and close the app and on again opening the app switch is once again turned OFF even thoe we left it turned ON while closing the app so to save this changes SharedPreferences.
Design:

code:
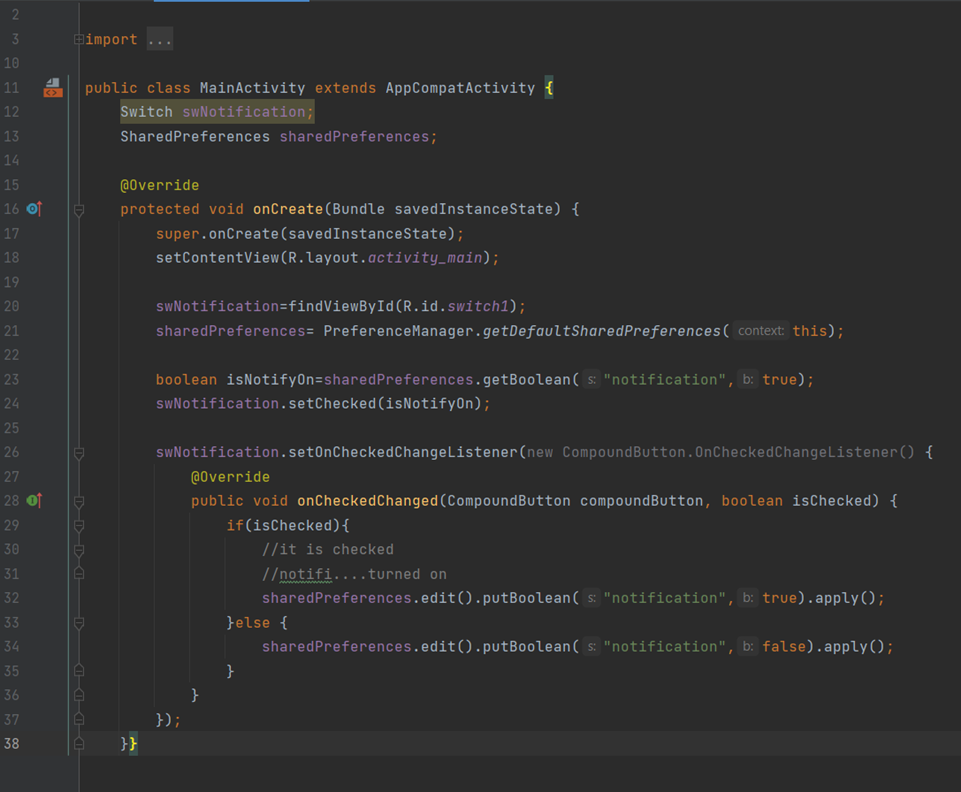
Android Switch (ON / OFF) Button
In android, Switch is a two-state user interface element that is used to display ON (Checked) or OFF (Unchecked) states as a button with thumb slider. By using thumb, the user may drag back and forth to choose an option either ON or OFF.
The Switch element is useful for the users to change the settings between two states either ON or OFF. We can add a Switch to our application layout by using Switch object.
Following is the pictorial representation of using Switch in android applications.
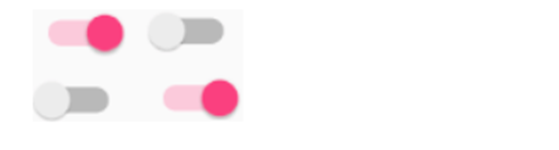
By default, the android Switch will be in the OFF (Unchecked) state. We can change the default state of Switch by using android:checked attribute.
In case, if we want to change the state of Switch to ON (Checked), then we need to set android:checked = “true” in our XML layout file.
In android, we can create Switch control in two ways either in the XML layout file or create it in the Activity file programmatically.
Create Switch Control in Activity File
In android, we can create Switch control programmatically in activity file based on our requirements. RelativeLayout layout = (RelativeLayout)findViewById(R.id.r_layout);
Switch sb = new Switch(this);
sb.setTextOff(“OFF”);
sb.setTextOn(“ON”);
sb.setChecked(true);
layout.addView(sb);
Handle Switch Click Events
Generally, whenever the user clicks on Switch, we can detect whether the Switch is in ON or OFF state and we can handle the Switch click event in activity file using setOnCheckedChangeListener like as shown below.
Custom SharedPreferences
When we use SharedPreferences extensively in our app, it’s advisable to create a dedicated class for them, specifically for accessing SharedPreferences. This way, we can call SharedPreferences functions whenever needed without having to create an object repeatedly.
class SharedPreferencesManager(context: Context) {
private val sharedPreferences: SharedPreferences = context.getSharedPreferences("MyPrefs", Context.MODE_PRIVATE)
private val editor: SharedPreferences.Editor = sharedPreferences.edit()
// Create or Update
fun saveString(key: String, value: String) {
editor.putString(key, value).apply()
}
// Read
fun getString(key: String, defaultValue: String = ""): String {
return sharedPreferences.getString(key, defaultValue) ?: defaultValue
}
// Update
fun updateString(key: String, newValue: String) {
if (sharedPreferences.contains(key)) {
editor.putString(key, newValue).apply()
}
}
// Delete
fun removeString(key: String) {
if (sharedPreferences.contains(key)) {
editor.remove(key).apply()
}
}
// Clear all data
fun clearAll() {
editor.clear().apply()
}
}
Database
Check out this : https://rishiz.site/to-do-note-app-in-android-sqlitedatabase/
[…] To understand SharePreference in details visit here. […]