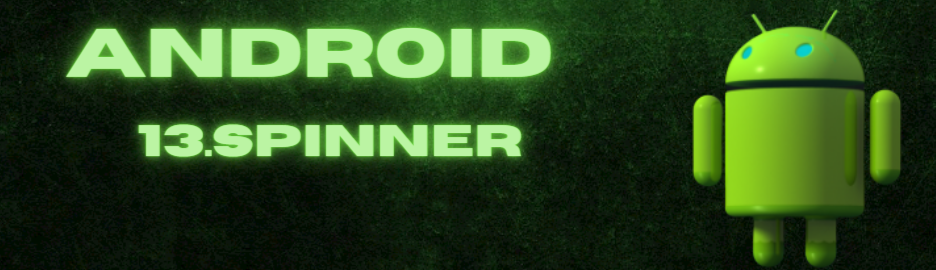
Creating Spinner in Android
Welcome to another edition of our development blog, where we delve into the world of Android development and explore various UI components. In this installment, we’re going to take a comprehensive look at the spinner in Android.
Table of Contents
What is an Android Spinner?
A spinner is a user interface element in Android that allows users to select a value from a predefined list of options. It is commonly used for presenting a set of choices, such as selecting a country, a category, or a date, from a dropdown menu. The selected value is displayed in a text field, and when the user taps the spinner, a dropdown menu appears, displaying the available options.

Implementation Steps
Step 1: Adding the Spinner to your Layout
To begin using the Spinner widget, you’ll need to add it to your XML layout file. Here’s a basic example:
<Spinner
android:id="@+id/spinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
Step 2: Populating the Spinner with Data
Once the Spinner is added to your layout, you’ll want to populate it with data. This is typically done using an adapter. Android provides various types of adapters, such as ArrayAdapter and CursorAdapter, depending on your data source.
Kotlin:
val spinner = findViewById<Spinner>(R.id.spinner)
val options: MutableList<String> = ArrayList()
options.add("Option 1")
options.add("Option 2")
options.add("Option 3")
val adapter = ArrayAdapter(this, android.R.layout.simple_spinner_item, options)
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item)
spinner.adapter = adapter
Java:
Spinner spinner = findViewById(R.id.spinner);
List<String> options = new ArrayList<>();
options.add("Option 1");
options.add("Option 2");
options.add("Option 3");
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, options);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
spinner.setAdapter(adapter);
In this example, we’re using an ArrayAdapter to populate the Spinner with simple text options. The setDropDownViewResource method sets the layout for the drop-down list of items.
Step 3: Handling Spinner Selection
Kotlin:
spinner.onItemSelectedListener = object : AdapterView.OnItemSelectedListener {
override fun onItemSelected(
parent: AdapterView<*>, view: View, position: Int, id: Long
) {
val selectedItem = parent.getItemAtPosition(position) as String
// Do something with the selected item
}
override fun onNothingSelected(parent: AdapterView<*>?) {
// Handle case where nothing is selected
}
}
Java:
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
String selectedItem = (String) parent.getItemAtPosition(position);
// Do something with the selected item
}
@Override
public void onNothingSelected(AdapterView<?> parent) {
// Handle case where nothing is selected
}
});
Creating a Custom Spinner in Android
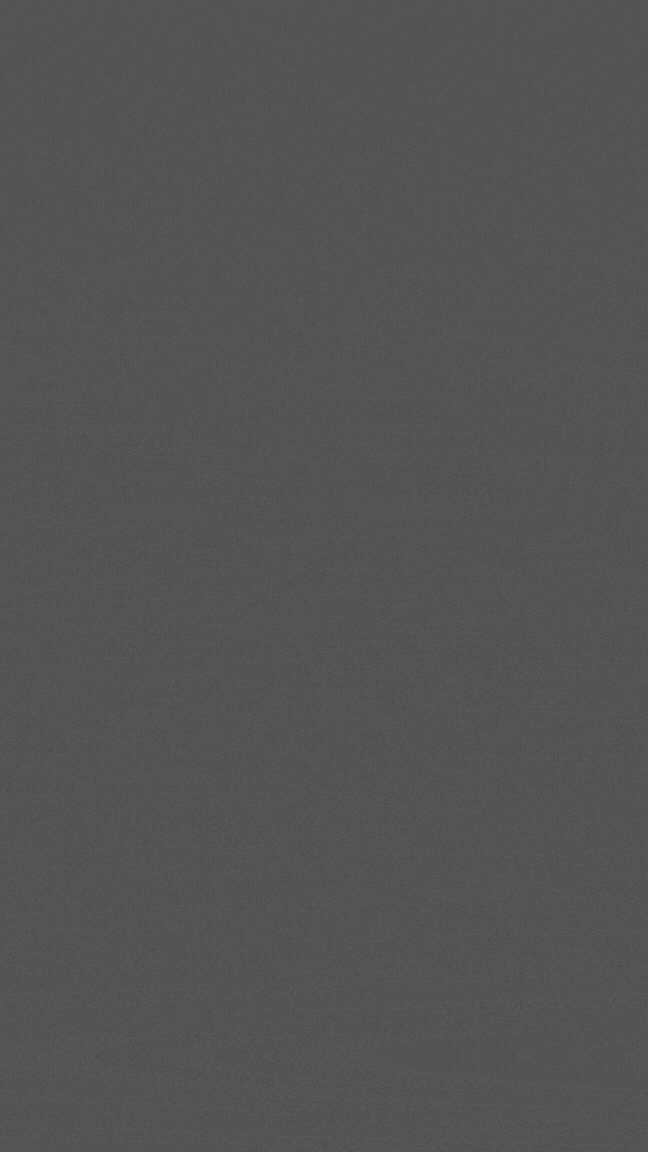
Step 1: Designing the Spinner Layout
In your app’s layout XML file (e.g., activity_main.xml
), define the spinner element. You can customize its appearance using attributes like layout_width
, layout_height
, and background
<Spinner
android:id="@+id/custom_spinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/spinner_background"
/>
Step 2: Creating the Custom Adapter
Create a custom adapter class that extends ArrayAdapter
. This adapter will provide data to populate the spinner items and define their appearance.
Kotlin:
class CustomSpinnerAdapter(private val context: Context, private val items: List<String>) :
ArrayAdapter<String?>(context, R.layout.custom_spinner_item, items) {
override fun getView(position: Int, convertView: View?, parent: ViewGroup): View {
val inflater = LayoutInflater.from(context)
val customView: View = inflater.inflate(R.layout.custom_spinner_item, parent, false)
val itemText = customView.findViewById<TextView>(R.id.item_text)
itemText.text = items[position]
return customView
}
override fun getDropDownView(position: Int, convertView: View, parent: ViewGroup): View {
val inflater = LayoutInflater.from(context)
val dropDownView: View =
inflater.inflate(R.layout.custom_spinner_dropdown_item, parent, false)
val itemText = dropDownView.findViewById<TextView>(R.id.dropdown_item_text)
itemText.text = items[position]
return dropDownView
}
}
Java:
public class CustomSpinnerAdapter extends ArrayAdapter<String> {
private Context context;
private List<String> items;
public CustomSpinnerAdapter(Context context, List<String> items) {
super(context, R.layout.custom_spinner_item, items);
this.context = context;
this.items = items;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
LayoutInflater inflater = LayoutInflater.from(context);
View customView = inflater.inflate(R.layout.custom_spinner_item, parent, false);
TextView itemText = customView.findViewById(R.id.item_text);
itemText.setText(items.get(position));
return customView;
}
@Override
public View getDropDownView(int position, View convertView, ViewGroup parent) {
LayoutInflater inflater = LayoutInflater.from(context);
View dropDownView = inflater.inflate(R.layout.custom_spinner_dropdown_item, parent, false);
TextView itemText = dropDownView.findViewById(R.id.dropdown_item_text);
itemText.setText(items.get(position));
return dropDownView;
}
}
Step 3: Designing Custom Item Layouts
Create two XML layout files, custom_spinner_item.xml
and custom_spinner_dropdown_item.xml
, in the res/layout
directory.
custom_spinner_item.xml
:
<TextView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/item_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16sp"
android:padding="8dp"
/>
custom_spinner_dropdown_item.xml
:
<TextView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/dropdown_item_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="16sp"
android:padding="8dp"
android:background="@drawable/spinner_dropdown_background"
/>
Step 4: Styling the Spinner
To make the custom spinner visually appealing, create appropriate background drawables. In the res/drawable
directory, add spinner_background.xml
and spinner_dropdown_background.xml
:
spinner_background.xml
(customize colors and shapes as desired):
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="#E0E0E0"/>
<corners android:radius="4dp"/>
</shape>
spinner_dropdown_background.xml
:
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="#FFFFFF"/>
<corners android:radius="4dp"/>
<stroke android:color="#AAAAAA" android:width="1dp"/>
</shape>
Step 5: Integrating the Custom Spinner
In your activity or fragment, initialize the custom spinner and set the custom adapter:
Kotlin:
class MainActivity : AppCompatActivity() {
private var customSpinner: Spinner? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
customSpinner = findViewById<Spinner>(R.id.custom_spinner)
val spinnerItems: MutableList<String> = ArrayList()
spinnerItems.add("Item 1")
spinnerItems.add("Item 2")
spinnerItems.add("Item 3")
val adapter = CustomSpinnerAdapter(this, spinnerItems)
customSpinner.setAdapter(adapter)
}
}
Java:
public class MainActivity extends AppCompatActivity {
private Spinner customSpinner;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
customSpinner = findViewById(R.id.custom_spinner);
List<String> spinnerItems = new ArrayList<>();
spinnerItems.add("Item 1");
spinnerItems.add("Item 2");
spinnerItems.add("Item 3");
CustomSpinnerAdapter adapter = new CustomSpinnerAdapter(this, spinnerItems);
customSpinner.setAdapter(adapter);
}
}
Step 6: Handling Spinner Selection
To respond to spinner item selection, you can set an OnItemSelectedListener
:
Kotlin:
customSpinner!!.onItemSelectedListener = object : AdapterView.OnItemSelectedListener {
override fun onItemSelected(
parentView: AdapterView<*>,
selectedItemView: View,
position: Int,
id: Long
) {
val selectedItem = parentView.getItemAtPosition(position) as String
// Handle the selected item
}
override fun onNothingSelected(parentView: AdapterView<*>?) {
// Handle the case where nothing is selected
}
}
Java:
customSpinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parentView, View selectedItemView, int position, long id) {
String selectedItem = (String) parentView.getItemAtPosition(position);
// Handle the selected item
}
@Override
public void onNothingSelected(AdapterView<?> parentView) {
// Handle the case where nothing is selected
}
});
Wrapping Up
And that’s a wrap on our exploration of the Android Spinner widget! Spinners are a versatile and efficient way to enhance user interactions in your Android apps. By understanding how to set up and customize them, you’ll be better equipped to provide seamless user experiences in your applications.