Listview in Android:Organized Data Presentation
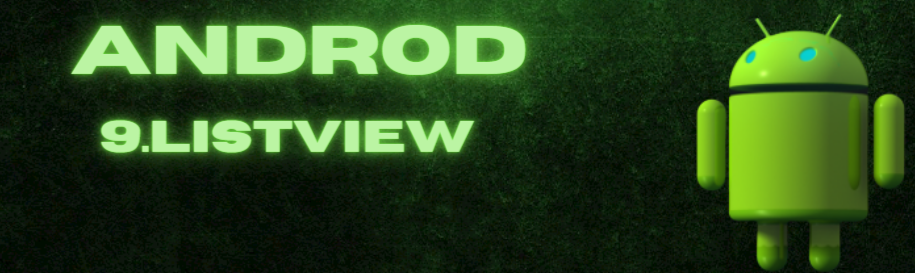
Efficiently presenting large datasets is a common challenge in the dynamic world of Android app development. That’s where the Android ListView comes in. This versatile and fundamental component allows developers to display lists of items in a structured and organized manner. In this blog post, we’ll Learn how to use the Android ListView to its fullest potential in your app.
Table of Contents
What is Android ListView?
Android ListView is a widget that efficiently displays a vertically scrollable list of items, where each item is a view element. It is particularly useful when you have a collection of data, such as a list of contacts, messages, or products, that you want to display in a structured manner to the user.
ListView is the subclass of AdapterView Class. The list items are inserted using an Adapter that pulls content from a source such as an array or a database.
Adapter converts an Array/Arraylist in to view items.
Implementing ListView
Implementing a ListView in your Android app can be done in a few simple steps, either programmatically using Java/Kotlin or via XML layout files
2. Define the Layout: Create a layout XML file that represents the appearance of each item in the list. This layout will be inflated and populated for each item in the ListView.
3. Prepare the Data: Gather the data you want to display in the list and organize it in a suitable data structure. Commonly used data structures are arrays, lists, or custom objects.
1. ListView in XML Layout:
4. Create the Adapter: Build a custom adapter by extending the ArrayAdapter or other available adapters like BaseAdapter or CursorAdapter
. The adapter acts as a bridge between your data and the ListView, responsible for converting the data into corresponding views for display.
5. Set the Adapter: Associate the adapter with your ListView using the setAdapter()
method.
Kotlin:
val adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, dataList)
val listView=findViewById<ListView>(R.id.listview)
listView.adapter = adapter
Java:
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, dataList); ListView listView = findViewById(R.id.listView); listView.setAdapter(adapter);
Example
Let’s take a look at an example.
Default Adapter

activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <ListView android:id="@+id/userlist" android:layout_width="match_parent" android:layout_height="wrap_content" > </ListView> </LinearLayout>
Kotlin:
MainActivity.kt
package net.rishiz.ktapp
import android.os.Bundle
import android.widget.ArrayAdapter
import android.widget.ListView
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
private val users = arrayOf(
"Ishwar Rathod",
"Rishi",
"Yash",
"Nikita",
"Kavita",
"Savita",
"Sumit",
"Sakshi",
"Rhys Herman",
"Alexis Patterson",
"Casey Fry",
"Marin Vega",
"Dale King",
"Xzavier Chang",
"Madav Sai",
" Yemineni",
"Rishi",
"Yash",
"Nikita",
"Kavita",
"Savita",
"Sumit",
"Sakshi"
)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, users)
val listView = findViewById<ListView>(R.id.listview)
listView.adapter = adapter
}
}
Java:
MainActivity.java
package rishiz.site.firstapp; import android.os.Bundle; import android.widget.ArrayAdapter; import android.widget.ListView; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { private final String[] users = {"Ishwar Rathod", "Rishi", "Yash", "Nikita", "Kavita", "Savita", "Sumit", "Sakshi", "Rhys Herman", "Alexis Patterson", "Casey Fry", "Marin Vega", "Dale King", "Xzavier Chang", "Madav Sai", " Yemineni", "Rishi", "Yash", "Nikita", "Kavita", "Savita", "Sumit", "Sakshi",}; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ListView mListView = (ListView) findViewById(R.id.userlist); ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, users); mListView.setAdapter(adapter); } }
Custom Adapters
For more complex data and custom layouts, you can create your own custom adapter by extending the BaseAdapter class. This allows you to have full control over the layout and data binding process.