JSON in Android
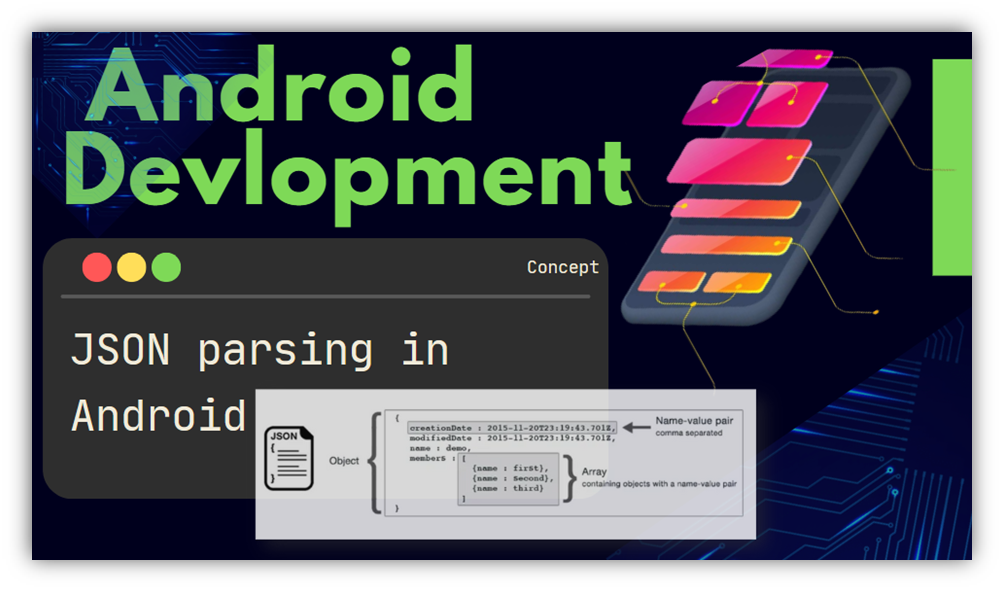
Table of Contents
What is the need for JSON
To know what is the need for JSON in Android or other platform first understand how server-based app works.
Client-server model how it works
Pretty much all of the app is server-based, which means that the majority of the data is stored on the server.
The benefit of data stored on the server:
1. As data is stored on the server it is more secure
2. the app will take up less space as data is stored on a server.
3. Multiple users can access data stored on one server.
If we need something, the app sends a request to the server for specific data. The server responds by sending the data.
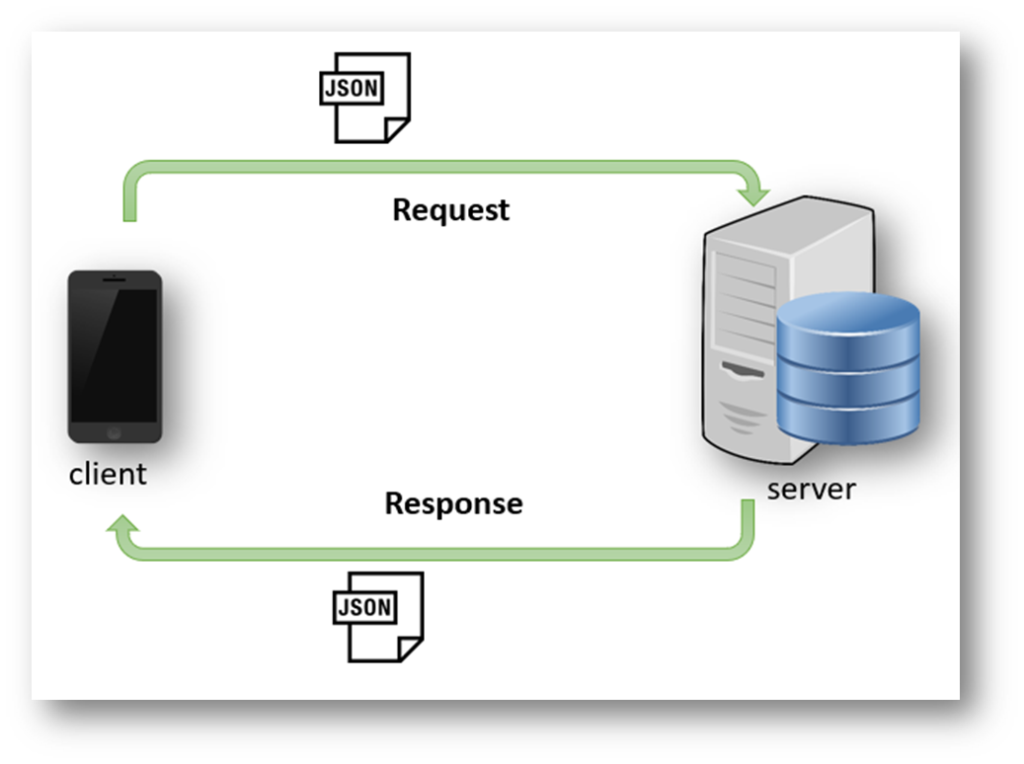
For example, let’s say you are using the Instagram app and you want to post an image.
To fulfill this requirement, the app will send a request object containing some other data, such as an image description. The server will then save the post in the database and send a response notifying you that the post has been uploaded successfully.
Now this way other users of this app can also see this post When users open the app or refresh the homepage, a request is sent to the server and the server responds with the most recent posts.
What is actually inside the server?
We call the server as backend(logic or brain) of our app it is something that the user can’t see.
The backend will consist of a database and a programming language such as PHP, Java, or Python. We use algorithms (logic) in these languages to store and retrieve data from the database. On the backend side, an endpoint refers to the code that executes on the server.
After we send a request, we hit specific endpoints on the server. Each endpoint is responsible for a particular action. For instance, if we want to post an image, we hit the endpoint that is in charge of posting images and saving them to the database.
Our request contains data that is extracted and processed on the server. After the server responds, the data is extracted on the app side.
This raises the question of how the backend and the app will be able to understand the data. For instance, if the backend is written in C# or PHP while our app is in Android(Java/Kotlin), how will the server be able to interpret the data we send to it? How can we achieve this kind of communication, especially since the iOS app is also using the same server?
To address this challenge, JSON comes into play. It is a widely used format for data exchange that can be interpreted by different programming languages. Regardless of the languages used for the backend and mobile app development, JSON can carry the data between them in a way that is easily understood.
Why only JSON
Let’s say there is an app to display information about pizzas.

But in what format can the data be transferred?
what about the plain text?

It is easy to read for us but not easy to interpret on the client side.
The XML was introduced, and it has been in use for many years, still, the format could be bulky and cause too much overhead for web services(network calls).
That is when the JSON came into play.
What is JSON
JSON stands for “JavaScript Object Notation.” It is a lightweight data-interchange format that is a lightweight format for storing and transporting data from the server to the app screen.
It is easy for humans to read and write and for machines to parse and generate.
JSON is a widely-used API output format that is independent of any programming language, despite its name, JavaScript Object Notation.
JSON represents data in two ways:
- Object: a collection of name-value (or key-value) pairs. An object is defined within left { and right } braces. Each name-value pair begins with the name, followed by a colon, followed by the value. Name-value paired are comma separated.
- Array: an ordered collection of values. An array is defined within left [ and right ] brackets. Items in the array are comma-separated.

- Keys: Every JSONObject has a key string that contains a certain value
- Value: Every key has a single value that can be of any type string, double, integer, boolean, etc
JSON files are commonly used in Android applications to transfer data from an API server. For instance, an API server usually returns a response in JSON format, so it is necessary to parse the response in JSON format into a Kotlin/Java object. Similarly, API servers also accept JSON format data. Therefore, to send it to an API server, Kotlin/Java objects must be parsed into JSON format.
Android provides four different classes to manipulate JSON data: JSONObject, JSONArray, JSONStringer and JSONTokenizer.
JSON parsing in Android
JSON Object
Create Textview and declare and initialize it.
Let’s assume you have the following JSON data:
{
“website”: {
“name”: “RISHIz”,
“Category”: “Android development”,
“post”:”json parsing”,
“post number”:9
}
}
Code:
package com.rishiz.app;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import org.json.JSONException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity {
public static final String JSON_STRING = "{\"Website\":{\"name\":\"RISHIz\",\"category\":\"Android development\",\"post\":\"json parsing\",\"post number\":9}}";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView textView = findViewById(R.id.textView2);
// Parsing the "Website" object
try {
JSONObject jsonObject = (new JSONObject(JSON_STRING)).getJSONObject("Website");
String name = jsonObject.getString("name");
String category = jsonObject.getString("category");
String post = jsonObject.getString("post");
String number = String.valueOf(jsonObject.getInt("post number"));
String str = "Website name:" + name + "\n" + "category:" + category + "\n" + "post:" + post + "\n" + "post number:" + number;
textView.setText(str);
} catch (JSONException e) {
throw new RuntimeException(e);
}
}
}
Output:

Parsing the nested “address” object
JSON Data:
{
"website": {
"name": "RISHIz",
"Category": "Android development",
"post":"json parsing",
"post number":9
“ Owner”:{
“name”:”Rushikesh Rathod”
“Experience”:”2 year”
}
}
Code:
package com.rishiz.app;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import org.json.JSONException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity {
public static final String JSON_STRING = "{\"Website\":{\"name\":\"RISHIz\",\"category\":\"Android development\",\"post\":\"json parsing\",\"post number\":9,"
+ "\"Owner\":{\"name\":\"Rushikesh Rathod\",\"Experience\":\"2 years\"}}}";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView textView = findViewById(R.id.textView2);
try {
// Parsing the "Website" object
JSONObject jsonObject = (new JSONObject(JSON_STRING)).getJSONObject("Website");
String name = jsonObject.getString("name");
String category = jsonObject.getString("category");
String post = jsonObject.getString("post");
String number = String.valueOf(jsonObject.getInt("post number"));
// Parsing the nested "Owner" object
JSONObject ownerObject = jsonObject.getJSONObject("Owner");
String ownerName = ownerObject.getString("name");
String ownerExperience = ownerObject.getString("Experience");
String str = "Website name:" + name + "\n" + "category:" + category + "\n" + "post:" + post + "\n"
+ "post number:" + number + "\n" + "Owner name:" + ownerName + "\n" + "Experience:" + ownerExperience;
textView.setText(str);
} catch (JSONException e) {
throw new RuntimeException(e);
}
}
}
Output:

JSON Array
JSON Data:
[ {"name":"RISHIz","url":"https://rishiz.site"}, {"name":"Linkedin","url":"https://www.linkedin.com/"}, {"name":"Github","url":"https://github.com"}]
Code:
package com.rishiz.app;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity {
public static final String JSON_STRING = "[ {\"name\":\"RISHIz\",\"url\":\"https://rishiz.site\"},{\"name\":\"Linkedin\",\"url\":\"https://www.linkedin.com/\"},{\"name\":\"Github\",\"url\":\"https://github.com\"}]";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = findViewById(R.id.btn);
btn.setOnClickListener(v -> {
try {
// Parsing the "JSONArray" object
JSONArray jsonArray = new JSONArray(JSON_STRING);
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject jsonObject = jsonArray.getJSONObject(i);
String name = jsonObject.getString("name");
String url = jsonObject.getString("url");
Log.d("JSON", "name " + i + ":" + name);
Log.d("JSON", "url " + i + ":" + url);
}
} catch (JSONException e) {
throw new RuntimeException(e);
}
});
}
}
Output:
Array in Object
JSON Data:
{
"fruits":[
"Apple",
"Apricot",
"Avocado",
"Banana",
"Bilberry",
"Blackberry"]}
Code:
package com.rishiz.app;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity {
public static final String JSON_ARRAY_STRING = "{\"fruits\":[\"Apple\",\"Apricot\",\"Avocado\",\"Banana\",\"Bilberry\",\"Blackberry\"]}";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = findViewById(R.id.btn);
btn.setOnClickListener(v -> {
try {
// Parsing the "JSONArray" object
JSONObject jsonObject = new JSONObject(JSON_ARRAY_STRING);
JSONArray jsonArray = jsonObject.getJSONArray("fruits");
for (int i = 0; i < jsonArray.length(); i++) {
String fruits = jsonArray.getString(i);
Log.d("JSON", "JSON_ARRAY Element " + i + ":" + fruits);
}
} catch (JSONException e) {
throw new RuntimeException(e);
}
});
}
}
we first create a JSONObject and then retrieve the JSONArray using this object because in JSON data ‘{‘ bracket is present at the start.
Output:
[…] Refer to this for the detailed working of networking: client-server-model-how-it-works […]